Introduction to gRPC on ASP.NET Core 3.0 (Part 1)

In preview3 of .NET Core 3.0 Microsoft showed us their implementation of the gRPC concepts which is kind of exciting and interesting. If you aren’t familiar with gRPC there’s a good reading on a subject here, below i will just provide shortened overview.
Very much alike WSDL/SOAP services, gRPC is all about calling remote methods over the Internet without worrying about platform or language that the service or the client is using. The nice part is that it doesn’t have all the downsides of the XML serialization/deserialization, when you mess with large blocks of XML, and processing it can imposes a huge performance penalty on the applications.
In gRPC we use something called protocol buffers. This is an exchange format and Interface Definition Language (IDL), which basically is a platform neutral mechanism to serialize structured data.
With protocol buffers, you write a .proto file with the description of the data structure you wish to store, then the protocol buffer compiler generates a class that implements automatic encoding and parsing of the protocol buffer data with an efficient binary format. This class is created with getters and setters for the fields and also takes care of reading and writing the protocol buffer as a unit.We cam also extend protocol buffer later over time in such a way that the code can still read data encoded with the old format.
Here’s some sample of .proto file i’m using in Part 2 of this series:
So, in our case the chosen language is C#, running the compiler on the above example will generate classes called JoinCustomerRequest, JoinCustomerReply, Customer, ChatMessage
and then these classes will be used in our application to populate, serialize, and retrieve corresponding protocol buffer messages. The payload of the messages is smaller than text format which is the default for RESTful services. So, in the end we’re getting more speed for the same amount of data transfered through the wire. And as a nice bonus, just by having this one .proto file you can generate both client and server code in many other languages, not just C#, but Go, C++, Java and other!
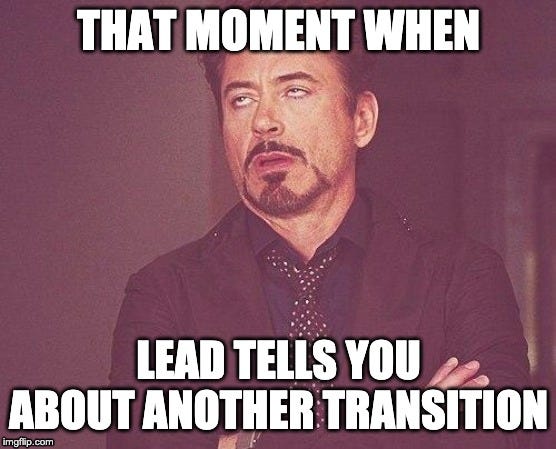
Now, we had WCF in the past, we have RESTful services with Web API 2 and here’s gRPC. A lot of solutions were written on enterprise level using either of the approaches and the question is do we need to rewrite everything? Do we need to switch again, this time to gRPC?
The answer to this is quite simple — it’s just another option in the toolkit and it’s completely up to the team to use gRPC or not. If you think about REST and gRPC without going deep into the philosophies these are basically different approaches to do the same thing. If you are starting something new and need to decide what approach to choose, then based on the information above, i’d think about the decision in a next way.
gRPC is a bit more prescriptive, basically — you define your data types and contracts first, design your service, design your service methods, parameters in your .proto file and then this is generated in the code. Which, probably, makes it not a bad choice for something to use within your network, something fast and something you can relatively quickly evolve and modify, especially if you like WCF. Connecting mobile devices to your backend services.
REST, on the other hand, is a solution for making an API that can be called by anybody in the world. It is a well known tech which is good if you goal to have a functionality that everybody can use and interoperate with.
In the Part 2 of this series we’ll go a little bit deeper into the C# code and implement simple service to manage customer information.
GL & HF